The coding implementation of interactive transcripts and PDF analysis is constructed through the LYZR CHATBOT framework

In this tutorial, we introduce a simplified method for extracting, processing, and analyzing YouTube video transcripts Lyzran advanced AI-driven framework designed to simplify interaction with text data. With Lyzr’s intuitive chatbot interface, together with YouTube-Transcript-API and FPDF, users can easily convert video content into structured PDF documents and conduct insightful analysis through dynamic interaction. Ideal for researchers, educators and content creators, Lyzr accelerates the process of gaining meaningful insights directly from multimedia resources, generating abstracts and raising creative questions.
!pip install lyzr youtube-transcript-api fpdf2 ipywidgets
!apt-get update -qq && apt-get install -y fonts-dejavu-core
We set up the necessary environment for the tutorial. The first command installed the required Python libraries, including Lyzr for AI-powered chat, YouTube-Transcript-API for transcripts, YouTube-Transcript-API for transcription extraction, FPDF2 for PDF generation, and IPYWIDGETS for creating interactive chat interfaces. The second command ensures that the DEJAVU SANS font is installed on the system to support full Unicode text rendering in the generated PDF file.
import os
import openai
openai.api_key = os.getenv("OPENAI_API_KEY")
os.environ['OPENAI_API_KEY'] = "YOUR_OPENAI_API_KEY_HERE"
We configure OpenAI API key access for the tutorial. We import the OS and OpenAI modules and then retrieve the API keys from the environment variables (or directly through the OS.Environ setting). This setup is critical to leveraging OpenAI’s powerful models within the LYZR framework.
import json
from lyzr import ChatBot
from youtube_transcript_api import YouTubeTranscriptApi, TranscriptsDisabled, NoTranscriptFound, CouldNotRetrieveTranscript
from fpdf import FPDF
from ipywidgets import Textarea, Button, Output, Layout
from IPython.display import display, Markdown
import re
View the full notebook here
We import the required libraries required for the tutorial. It includes JSON for data processing, AI-driven chat capabilities of LYZR’s chatbot, and YouTubetransscriptapi for extracting transcripts from YouTube videos. Additionally, it generates PDF, IPYWIDGETS provides FPDF for interactive UI components and IPYTHON.DISPLAY for rendering Markdown content in notebooks. The RE module is also used for regular expression operations in text processing tasks.
def transcript_to_pdf(video_id: str, output_pdf_path: str) -> bool:
"""
Download YouTube transcript (manual or auto) and write it into a PDF
using the system-installed DejaVuSans.ttf for full Unicode support.
Fixed to handle long words and text formatting issues.
"""
try:
entries = YouTubeTranscriptApi.get_transcript(video_id)
except (TranscriptsDisabled, NoTranscriptFound, CouldNotRetrieveTranscript):
try:
entries = YouTubeTranscriptApi.get_transcript(video_id, languages=['en'])
except Exception:
print(f"[!] No transcript for {video_id}")
return False
except Exception as e:
print(f"[!] Error fetching transcript for {video_id}: {e}")
return False
text = "n".join(e['text'] for e in entries).strip()
if not text:
print(f"[!] Empty transcript for {video_id}")
return False
pdf = FPDF()
pdf.add_page()
font_path = "/usr/share/fonts/truetype/dejavu/DejaVuSans.ttf"
try:
if os.path.exists(font_path):
pdf.add_font("DejaVu", "", font_path)
pdf.set_font("DejaVu", size=10)
else:
pdf.set_font("Arial", size=10)
except Exception:
pdf.set_font("Arial", size=10)
pdf.set_margins(20, 20, 20)
pdf.set_auto_page_break(auto=True, margin=25)
def process_text_for_pdf(text):
text = re.sub(r's+', ' ', text)
text = text.replace('nn', 'n')
processed_lines = []
for paragraph in text.split('n'):
if not paragraph.strip():
continue
words = paragraph.split()
processed_words = []
for word in words:
if len(word) > 50:
chunks = [word[i:i+50] for i in range(0, len(word), 50)]
processed_words.extend(chunks)
else:
processed_words.append(word)
processed_lines.append(' '.join(processed_words))
return processed_lines
processed_lines = process_text_for_pdf(text)
for line in processed_lines:
if line.strip():
try:
pdf.multi_cell(0, 8, line.encode('utf-8', 'replace').decode('utf-8'), align='L')
pdf.ln(2)
except Exception as e:
print(f"[!] Warning: Skipped problematic line: {str(e)[:100]}...")
continue
try:
pdf.output(output_pdf_path)
print(f"[+] PDF saved: {output_pdf_path}")
return True
except Exception as e:
print(f"[!] Error saving PDF: {e}")
return False
View the full notebook here
This feature, Trascript_to_pdf, automatically converts YouTube video transcripts into clean, readable PDF documents. It uses YouTube Etransscriptapi to retrieve transcripts, handles exceptions gracefully, such as unavailable transcripts, and formats text to avoid problems like breaking PDF layout. The function can also ensure proper Unicode support by using Dejavusans fonts (if any), and optimize PDF rendered text by splitting too long words and maintaining consistent margins. Return true if PDF or error is successfully generated.
def create_interactive_chat(agent):
input_area = Textarea(
placeholder="Type a question…", layout=Layout(width="80%", height="80px")
)
send_button = Button(description="Send", button_style="success")
output_area = Output(layout=Layout(
border="1px solid gray", width="80%", height="200px", overflow='auto'
))
def on_send(btn):
question = input_area.value.strip()
if not question:
return
with output_area:
print(f">> You: {question}")
try:
print("
View the full notebook here
This feature, create_interactive_chat, creates a simple and interactive chat interface in Colab. Using iPyWidgets provides a text input area (TextArea) for users to enter questions, send buttons (buttons) to trigger chat and output areas (output) to display conversations. When the user clicks Send, the entered question is passed to the LYZR CHATBOT proxy, which generates and displays a response. This enables users to conduct dynamic Q&A sessions based on transcript analysis, making it the same as real-time conversations with AI models.
def main():
video_ids = ["dQw4w9WgXcQ", "jNQXAC9IVRw"]
processed = []
for vid in video_ids:
pdf_path = f"{vid}.pdf"
if transcript_to_pdf(vid, pdf_path):
processed.append((vid, pdf_path))
else:
print(f"[!] Skipping {vid} — no transcript available.")
if not processed:
print("[!] No PDFs generated. Please try other video IDs.")
return
first_vid, first_pdf = processed[0]
print(f"[+] Initializing PDF-chat agent for video {first_vid}…")
bot = ChatBot.pdf_chat(
input_files=[first_pdf]
)
questions = [
"Summarize the transcript in 2–3 sentences.",
"What are the top 5 insights and why?",
"List any recommendations or action items mentioned.",
"Write 3 quiz questions to test comprehension.",
"Suggest 5 creative prompts to explore further."
]
responses = {}
for q in questions:
print(f"[?] {q}")
try:
resp = bot.chat(q)
except Exception as e:
resp = f"[!] Agent error: {e}"
responses[q] = resp
print(f"[/] {resp}n" + "-"*60 + "n")
with open('responses.json','w',encoding='utf-8') as f:
json.dump(responses,f,indent=2)
md = "# Transcript Analysis Reportnn"
for q,a in responses.items():
md += f"## Q: {q}n{a}nn"
with open('report.md','w',encoding='utf-8') as f:
f.write(md)
display(Markdown(md))
if len(processed) > 1:
print("[+] Generating comparison…")
_, pdf1 = processed[0]
_, pdf2 = processed[1]
compare_bot = ChatBot.pdf_chat(
input_files=[pdf1, pdf2]
)
comparison = compare_bot.chat(
"Compare the main themes of these two videos and highlight key differences."
)
print("[+] Comparison Result:n", comparison)
print("n=== Interactive Chat (Video 1) ===")
create_interactive_chat(bot)
View the full notebook here
Our Main() function is the core driver for the entire tutorial pipeline. It processes a list of YouTube video IDs and uses the Transcript_TO_PDF function to convert available transcripts into PDF files. After the PDF is generated, the LYZR PDF-CHAT proxy is initialized on the first PDF, allowing the model to answer predefined questions such as aggregating content, identifying insights, and generating quiz questions. The answer is stored in the response. JSON file and formatted as Markdown Report (Report.MD). If multiple PDFs are created, the feature is compared using the Lyzr proxy to highlight the key differences between the videos. Finally, it launches an interactive chat interface with the user, enabling dynamic conversations based on transcript content, demonstrating Lyzr’s capabilities for seamless PDF analytics and AI-driven interactions.
if __name__ == "__main__":
main()
We make sure that the script will only run when it is directly executed, i.e. when it is imported as a module. In Python scripts, this is the best practice for controlling execution flow.
In short, by integrating Lyzr into the workflow shown in this tutorial, we can easily convert YouTube videos into insightful, actionable knowledge. Lyzr’s intelligent PDF-CHAT feature simplifies extracting core topics and generating comprehensive summary, and also allows for engaging and interactive exploration of content through an intuitive dialogue interface. Adopting Lyzr allows users to unlock deeper insights and significantly improve productivity when using video transcripts, whether for academic research, educational purposes or creative content analysis.
Check out the notebook here. All credits for this study are to the researchers on the project. Also, please stay tuned for us twitter And don’t forget to join us 95k+ ml reddit And subscribe Our newsletter.
Asif Razzaq is CEO of Marktechpost Media Inc. As a visionary entrepreneur and engineer, ASIF is committed to harnessing the potential of artificial intelligence to achieve social benefits. His recent effort is to launch Marktechpost, an artificial intelligence media platform that has an in-depth coverage of machine learning and deep learning news that can sound both technically, both through technical voices and be understood by a wide audience. The platform has over 2 million views per month, demonstrating its popularity among its audience.
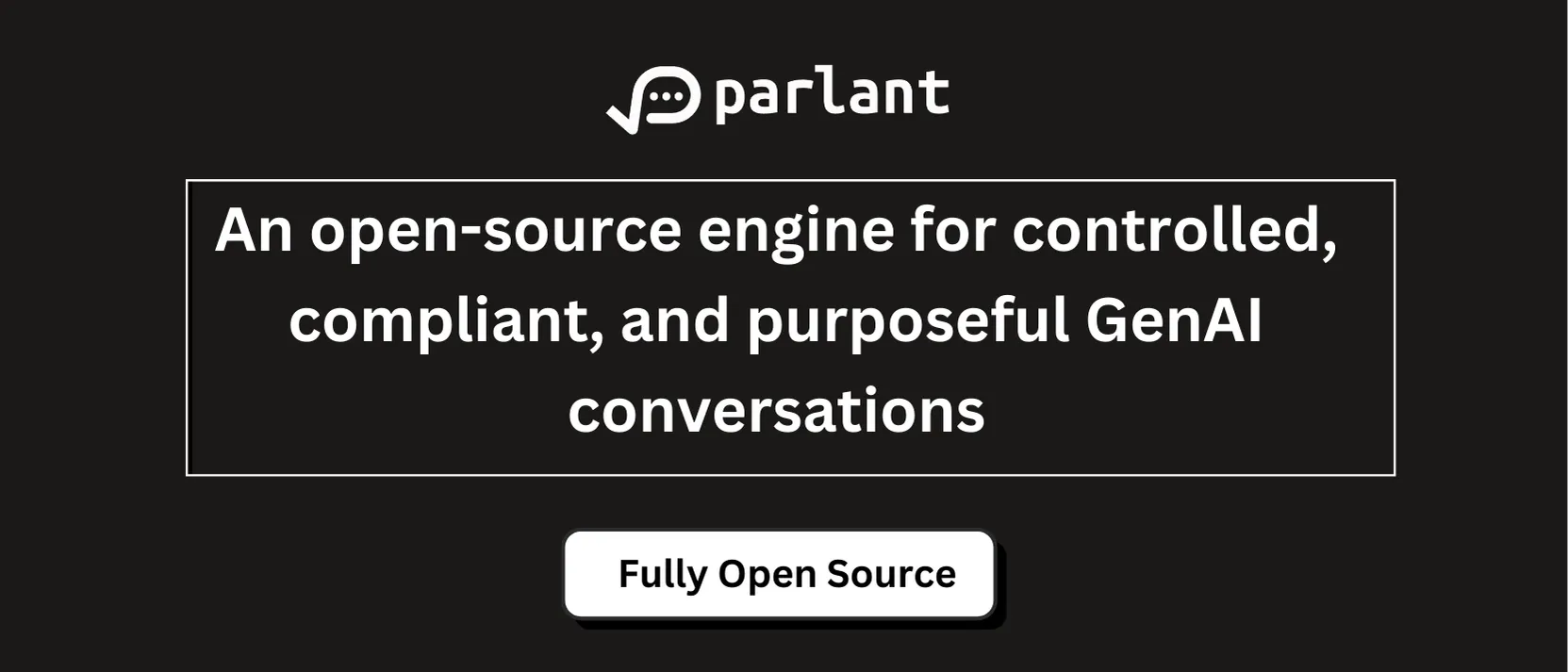